You can programmatically open SAP GUI from VBA by utilizing the sapshcut.exe executable, typically found in the SAP installation directory.
The default SAP installation directory is "C:\Program Files (x86)\SAP\FrontEnd\SAPgui\".
Please note that this method only works when logging in to SAP through the traditional method where you have a username and password, and not via Single Sign On (SSO).
I have not yet found a reliable way to automate SSO login through VBA.
Obtaining Required Arguments for sapshcut.exe
To run sapshcut.exe, four arguments are required: system name, client number, SAP username, and password. You can retrieve the system name and client number in SAP sessions at the bottom right corner. You can refer to the diagram below to locate the information:
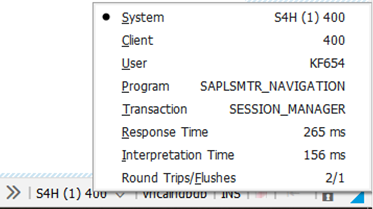
Once you got the SAP system name and client number, input these details into the following VBA function. Replace the placeholders with your actual system name, client number, username, and password and you will be able to login to SAP by calling the function.
Function RunSAP(system As String, client As String, user As String, pw As String)
Dim command As String
command = "C:\Program Files (x86)\SAP\FrontEnd\SAPgui\sapshcut.exe -system=" & system & " -client=" & client & " -user=" & user & " -pw=" & pw & " -language=en"
Shell command, vbNormalFocus
End Function
Similar script can also be ran using Python. If you are using Python, you can use the following python script:
def start_sap():
command = [
"C:\\Program Files (x86)\\SAP\\FrontEnd\\SAPgui\\sapshcut.exe",
"-system=S4H",
"-client=400",
"-user=<your_username>",
"-pw=<your_password>",
"-language=en"
]
subprocess.run(command, shell=True)
When implementing this function, remember that you must check if SAP has finished loading before executing your SAP GUI script. Failing to do so will result in errors, as the connection to SAP cannot be established until the system has fully loaded.
Ensure you include this crucial check to avoid any issues and guarantee a seamless execution of your script.
Learning SAP GUI Scripting as a Beginner
If you are eager to unlock the power of SAP GUI Scripting, I've got you covered! I've written a comprehensive eBook that covers everything you need to know to get started.
With this eBook, you'll gain a complete understanding of the SAP GUI object model and get access to practical code snippets that will help you hit the ground running.
Get your copy now and start your SAP GUI Scripting journey today!